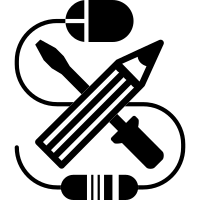
I'd rather reserve the package for components that have proven repeatedly useful, like `Classes` and `HTML5`.
65 lines
1.6 KiB
Go
65 lines
1.6 KiB
Go
// Package components provides high-level components and helpers that are composed of low-level elements and attributes.
|
|
package components
|
|
|
|
import (
|
|
"io"
|
|
"sort"
|
|
"strings"
|
|
|
|
g "github.com/maragudk/gomponents"
|
|
. "github.com/maragudk/gomponents/html"
|
|
)
|
|
|
|
// HTML5Props for HTML5.
|
|
// Title is set no matter what, Description and Language elements only if the strings are non-empty.
|
|
type HTML5Props struct {
|
|
Title string
|
|
Description string
|
|
Language string
|
|
Head []g.Node
|
|
Body []g.Node
|
|
}
|
|
|
|
// HTML5 document template.
|
|
func HTML5(p HTML5Props) g.Node {
|
|
return Doctype(
|
|
HTML(g.If(p.Language != "", Lang(p.Language)),
|
|
Head(
|
|
Meta(Charset("utf-8")),
|
|
Meta(Name("viewport"), Content("width=device-width, initial-scale=1")),
|
|
TitleEl(g.Text(p.Title)),
|
|
g.If(p.Description != "", Meta(Name("description"), Content(p.Description))),
|
|
g.Group(p.Head),
|
|
),
|
|
Body(g.Group(p.Body)),
|
|
),
|
|
)
|
|
}
|
|
|
|
// Classes is a map of strings to booleans, which Renders to an attribute with name "class".
|
|
// The attribute value is a sorted, space-separated string of all the map keys,
|
|
// for which the corresponding map value is true.
|
|
type Classes map[string]bool
|
|
|
|
func (c Classes) Render(w io.Writer) error {
|
|
var included []string
|
|
for c, include := range c {
|
|
if include {
|
|
included = append(included, c)
|
|
}
|
|
}
|
|
sort.Strings(included)
|
|
return Class(strings.Join(included, " ")).Render(w)
|
|
}
|
|
|
|
func (c Classes) Type() g.NodeType {
|
|
return g.AttributeType
|
|
}
|
|
|
|
// String satisfies fmt.Stringer.
|
|
func (c Classes) String() string {
|
|
var b strings.Builder
|
|
_ = c.Render(&b)
|
|
return b.String()
|
|
}
|