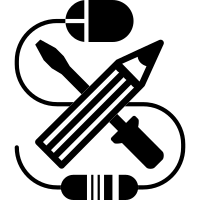
* remove submodules * add api and ui files * update github actions * use sparse checkout * update node setup * update checkout * update docker * change permissions * update mariadb health check * update changelog
30 lines
731 B
TypeScript
30 lines
731 B
TypeScript
import { useEffect, useState } from 'react'
|
|
|
|
export const useWindowSize = (): { width?: number; height?: number } => {
|
|
const isClient = typeof window === 'object'
|
|
|
|
function getSize() {
|
|
return {
|
|
width: isClient ? window.innerWidth : undefined,
|
|
height: isClient ? window.innerHeight : undefined,
|
|
}
|
|
}
|
|
|
|
const [windowSize, setWindowSize] = useState(getSize)
|
|
|
|
useEffect(() => {
|
|
if (!isClient) {
|
|
return
|
|
}
|
|
|
|
function handleResize() {
|
|
setWindowSize(getSize())
|
|
}
|
|
|
|
window.addEventListener('resize', handleResize)
|
|
return () => window.removeEventListener('resize', handleResize)
|
|
}, []) // Empty array ensures that effect is only run on mount and unmount
|
|
|
|
return windowSize
|
|
}
|