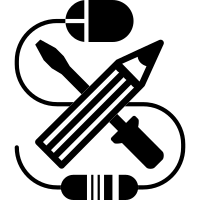
* remove submodules * add api and ui files * update github actions * use sparse checkout * update node setup * update checkout * update docker * change permissions * update mariadb health check * update changelog
54 lines
1.3 KiB
TypeScript
54 lines
1.3 KiB
TypeScript
import { QueryHookOptions, QueryResult, useQuery } from '@apollo/client'
|
|
import { gql } from '@apollo/client/core'
|
|
import { useImperativeQuery } from '../../components/use.imerative.query'
|
|
import { FORM_PAGER_FRAGMENT, FormPagerFragment } from '../fragment/form.pager.fragment'
|
|
import { SUBMISSION_FRAGMENT, SubmissionFragment } from '../fragment/submission.fragment'
|
|
|
|
interface Data {
|
|
pager: {
|
|
entries: SubmissionFragment[]
|
|
|
|
total: number
|
|
limit: number
|
|
start: number
|
|
}
|
|
|
|
form: FormPagerFragment
|
|
}
|
|
|
|
interface Variables {
|
|
form: string
|
|
start?: number
|
|
limit?: number
|
|
filter?: {
|
|
finished?: boolean
|
|
excludeEmpty?: boolean
|
|
}
|
|
}
|
|
|
|
const QUERY = gql`
|
|
query listSubmissions($form: ID!, $start: Int, $limit: Int, $filter: SubmissionPagerFilterInput) {
|
|
form: getFormById(id: $form) {
|
|
...PagerForm
|
|
}
|
|
|
|
pager: listSubmissions(form: $form, start: $start, limit: $limit, filter: $filter) {
|
|
entries {
|
|
...Submission
|
|
}
|
|
total
|
|
limit
|
|
start
|
|
}
|
|
}
|
|
|
|
${SUBMISSION_FRAGMENT}
|
|
${FORM_PAGER_FRAGMENT}
|
|
`
|
|
|
|
export const useSubmissionPagerQuery = (
|
|
options?: QueryHookOptions<Data, Variables>
|
|
): QueryResult<Data, Variables> => useQuery<Data, Variables>(QUERY, options)
|
|
|
|
export const useSubmissionPagerImperativeQuery = () => useImperativeQuery<Data, Variables>(QUERY)
|