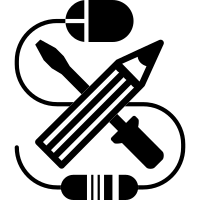
* remove submodules * add api and ui files * update github actions * use sparse checkout * update node setup * update checkout * update docker * change permissions * update mariadb health check * update changelog
29 lines
793 B
TypeScript
29 lines
793 B
TypeScript
import { createWrapper, HYDRATE } from 'next-redux-wrapper'
|
|
import { AnyAction, applyMiddleware, combineReducers, createStore, Store } from 'redux'
|
|
import { composeWithDevTools } from 'redux-devtools-extension'
|
|
import thunkMiddleware from 'redux-thunk'
|
|
import { auth, AuthState } from './auth'
|
|
|
|
export interface State {
|
|
auth: AuthState
|
|
}
|
|
|
|
const root = (state: State, action: AnyAction): State => {
|
|
switch (action.type) {
|
|
case HYDRATE:
|
|
return { ...state, ...action.payload } as State
|
|
}
|
|
|
|
const combined = combineReducers({
|
|
auth,
|
|
})
|
|
|
|
return combined(state, action)
|
|
}
|
|
|
|
const makeStore = () => {
|
|
return createStore(root, undefined, composeWithDevTools(applyMiddleware(thunkMiddleware)))
|
|
}
|
|
|
|
export const wrapper = createWrapper<Store<State>>(makeStore, { debug: false })
|